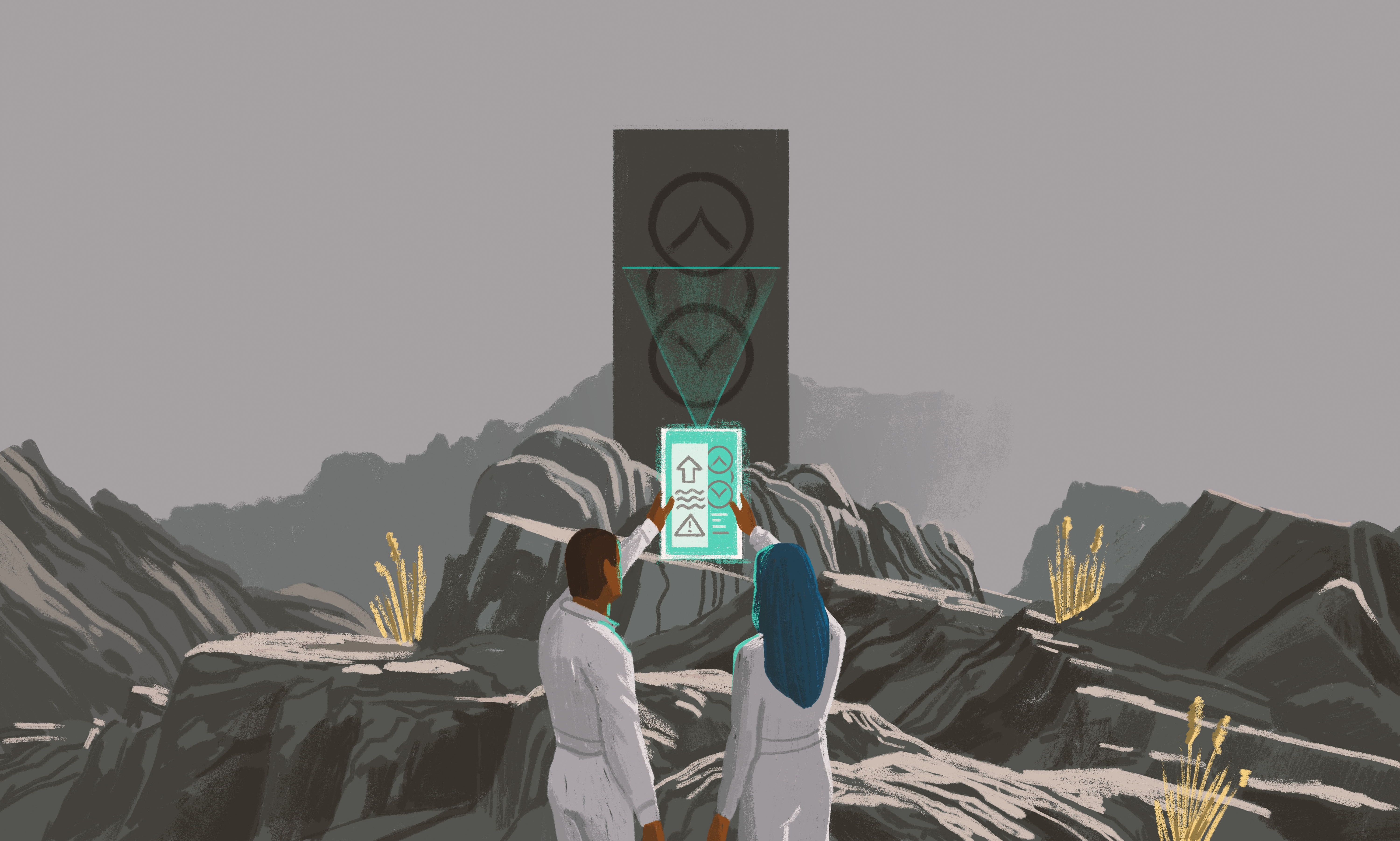
In an attempt to be "accessible" most developers tend to add ARIA all throughout their code without realizing how detrimental this can actually be for those that rely on this tool. Before one starts adding ARIA to their code, it is important to understand exactly what it is, why it is used, and how it can impact a user.
What is ARIA
“WAI-ARIA”, stands for Web Accessibility Initiative - Accessible Rich Internet Applications, and tends to be referred to by its shortened name, “ARIA”. ARIA enhances assistive technology’s interaction of web pages in ways that plain HTML cannot do, by giving more context to dynamic content and advanced UI controls. It is important to note that ARIA is only used to convey information to assistive technology users, it does not change or inject functionally into the code for a user’s experience. It is made for assistive technology users to gather more information about what is happening on the page and what to expect when they interact with an element. It is up to the developer to ensure the proper naming conventions are conveyed to the user and the appropriate functionality is created and works as expected. It is important to remember that classes, ID’s, and any visual styling like color, bold, italics, etc.is not communicated to a screen reader user, so we convey this information in a different way, using semantic HTML and ARIA.
ARIA is only used to convey information to assistive technology users, it does not change or inject functionally into the code for a user’s experience.
Roles, States, and Properties
ARIA is made up of 3 main components: roles, states, and properties. Including these within your code helps communicate to an assistive tech user what an element is, what it is going to do, and the current state it is in.
Roles
ARIA roles define what an element is or does and are designated by the role
attribute. This role attribute announces an element to a user that doesn’t exist in HTML semantics or to expand on semantic HTML elements.
Some common examples of ARIA roles are:
role="menu" role="menuitem" role="tab" role="tablist" role="tabpanel" role="presentation" role="alert" role="marquee"
Important notes to know about ARIA role:
- Some roles require parent roles to convey the correct information. An example of this is
role="tab"
androle="tablist"
.role="tab"
can only be used if the parent wrapper has thetablist
role attached to it. - Just because you add ARIA roles to your code, it does not change the functionality. You are still responsible for adding in the correct JavaScript code to implement the expected functionality of a
tablist
for example.
States and Properties
Both States and Properties convey information to the user about what to expect or what is currently happening with the interaction or relationship of the element. Using that role="tab"
example, this is a great way to convey to the user which visual tab is currently active and visible.
- States: Attributes that define the current condition or state of an element.
- Properties: Define additional semantics not supported in standard HTML.
Some common examples of ARIA states and properties are:
aria-haspopup="true" aria-expanded="false" aria-required="true" aria-live="polite" aria-labelledy="#ofElement" aria-current="page"
The five rules of ARIA
Now that you have a good background and basic understanding of what makes up ARIA, it is time to understand the five rules of ARIA.
1 | Do not use ARIA if you can use a native HTML element or attribute instead
ARIA overrides the original HTML semantics or text content that is conveyed to assistive technology.
ARIA just conveys information to a user, it does not change functionality. Adding ARIA to change semantics conveys the new role to the screen reader and not the original HTML semantic element.
If you find you are adding a different role than what the native element is, then it should probably just be the correct semantic element to begin with.
Assistive technology users expect consistent interaction patterns and audio feedback from components.
It is important to remember that assistive technology users require this technology to properly interact with your page. We must follow the expected patterns and audio feedback users were taught when interacting with components. For example, if someone is interacting with a checkbox, they expect to hear if the checkbox is checked - or not - upon selection.
Going outside of these norms can be detrimental to the user’s experience. Think of it as if someone sent you a webpage and now you have to read from right to left, when your native language reads from left to right. You could learn to do this, but do you want to? Probably not. You will go elsewhere to find this information.
2 | Do not change native semantics unless you really (really, really, really) have to
When can I change native HTML with ARIA? Well, almost never. Writing this, it actually took me some serious time to come up with an example where it is appropriate to override native semantics with ARIA. The only time I would recommend this is when creating an email template.
For those that don’t know, email templates still need to be coded within a table structure. One of the first rules of accessible code is to not use tables to structure content and to only use it for tabular data. However, in this case, it is impossible to do. By adding role=presentation
to the HTML table, it removes the table structure from being announced to the screen reader users, and leaves just the content within the table to be announced.
3 | All interactive ARIA controls must be usable with the keyboard
If you create a custom widget that a user can click or tap or drag or drop or slide or scroll, a user must also be able to navigate to that same widget, perform an equivalent action and get the same result using just the keyboard.
All interactive widgets must be scripted to respond to standard keystrokes or keystroke combinations where applicable. This is because all assistive technology actually uses these keystrokes to interact in the back end, even if the user’s tech is not keyboard input.
And of course, not only must it work via keyboard, but it must also follow the expected patterns an assistive technology user would expect as we talked about in rule #1.
It is important to remember that ARIA has nothing to do with keyboard functionality, but rather it provides the semantics to the accessibility APIs. That being said, it is the developer’s responsibility to make any custom controls accessible with the keyboard by using Javascript and other coding techniques. And that’s where rule #1 comes back into play again - and why it is important to use native HTML elements!
4 | Do not use role=presentation
or aria-hidden=true
on a focusable element
This is another great example where ARIA is just conveying information to a user, but it doesn’t change how it functions. If you add aria-hidden=true
to an element, especially an interactive element, a user will still see the element, but will not be able to interact with it at all. It is important to remember that not all screen reader users are non sighted, so we want to make sure the visuals line up with what the user is hearing.
5 | All interactive elements must have an accessible name
An accessible name means that there is a label or name that the accessibility API property has a value. I always say this as a “programmatic” name. And basically it is what is conveyed to a screen reader or other assistive tech user when interacting with the element. Ideally the accessible name and the visual text match, but sometimes the accessible name has more text to allow for more context.
You may be asking - how do I know what the accessible name is or see this accessibility API to confirm? Firefox does a great job of displaying what the accessible name of an element is. Open up the Firefox web developer tools, go to the “Accessibility” tab, and once you highlight the element, it will show you the “name” in the right column. If this says “null”, then the accessible name is missing.
Examples of what is considered an accessible name:
- Content or text within an element
aria-label
aria-labelledby
- Title within an SVG
- Alt text on an image
- Label on an input
And of course, the most important rule of using ARIA is:
No ARIA is Better than Bad ARIA
ARIA can be very helpful to users who rely on it, but only when it is implemented correctly. It is better to use no ARIA in your code at all, and to rely solely on normal HTML semantics, than to include too much or incorrect ARIA. Taking a step back to rethink your use of ARIA and following the five rules when creating your code will help to ensure your code is done well and is accessible to all.
If you are interested in learning more about using WAI-ARIA correctly, check out my recent webinar, Do More With Less Aria.