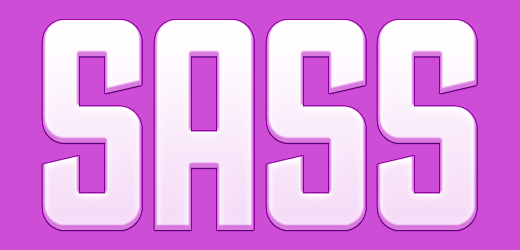
A while ago we (Joel) published an article titled, “LESS, The Flavor of CSS You Should be Writing for Drupal.” At the time (2010), preprocessors were just starting to gain popularity. LESS seemed great and there was a Drupal module for compiling on the server. So, we switched from CSS to LESS. It was fantastic. Variables, mixins, nested rules; everything we ever wanted in CSS.
A year or so later the talk around SASS picked up. We hadn’t considered it as an option when first exploring preprocessors because SASS 3 wasn’t released yet. SASS 3 is notable because it marked the introduction of the .scss syntax (a syntax that more closely mirrors CSS). On top of a more familiar syntax, SASS 3 brought the @extend
directive. This was the thing that made me excited (I’ll talk more about that later). It was clear that SASS was outpacing LESS when it came to features. So, we switched again.
I’m not going to get into a SASS vs. LESS comparison here. Chris Coyier recently published a fantastic article, “SASS vs. LESS,” comparing the two preprocessors. Instead, I want to cover the three features that should convince you to use SASS over anything else.
This was the thing that made us switch from LESS to SASS. Let’s take a look at some CSS and see how powerful @extend
can be.
First, here’s are two simple “mixins” (Supported in both SASS and LESS):
With these you can easily apply that CSS across a number of elements on the site like this:
which becomes:
Awesome! Oh wait, is that really awesome? Doesn’t this lead to a lot of redundant CSS? What if we want to use that CSS all over the place? What if that mixin has 10 lines of CSS instead of just 1? That CSS gets added for every time you include the mixin. Yow! You can see how this could quickly lead to huge stylesheets. Now let’s look at @extend
.
Instead of mixins, let’s write some selectors. In this case, we’re going to use a new feature in SASS 3.2 alpha called “placeholder selectors.” Instead of using the “.selector
” syntax, they use “%selector
” which tells SASS not to render the base selector name. Here we go:
Then we use @extend
to apply the styles like so:
Which gives us this:
“Hold on. That’s not better!” is what you’re probably saying. First, settle down. Let me explain.
Ok, ready? Let’s look at a more complex example.
Now we can write:
Which compiles to:
Now that is cool! Instead of including those four lines of CSS each time, the selectors were joined together for more condensed, modular CSS. And this is just a simple example. The possibilities here are endless. (Quick note: This is not an excuse to just target any class or id and extend CSS rules. Check out SMACSS for some guidance on structuring your CSS.)
You know what’s hot? Responsive design. SASS makes it dead simple to work with @media queries in your CSS. Here’s a quick example:
which becomes:
Let’s take this a step further and include Sam Richard’s respond-to
mixin (which can be found here: https://gist.github.com/2493551). This will create the same code as the previous example but with a shorter, easier to remember syntax. If you ever need to adjust things later, you just have to do it in one place.
Finally, we can’t talk about SASS without talking about Compass. Compass is a CSS framework that will save you countless hours. COUNTLESS! Let’s talk about how.
Have you ever manually written all of the vendor-prefixed properties for border-radius or all the other CSS3 properties? Yeah, Compass does that for you.
How about gradients? They can be pretty tricky. With Compass they’re cake (http://compass-style.org/reference/compass/css3/images/).
Do you design with a baseline grid? Well, Compass has oodles of mixins to help create a consistent vertical rhythm. (http://compass-style.org/reference/compass/typography/vertical_rhythm/).
I've covered a lot here but this is just a taste of what's possible with SASS and Compass. If you haven’t tried a preprocessor yet, do it. Right now! If you’re not sure what to use, use SASS. Try it and I guarantee you’ll realize within minutes how powerful it is and how much time you’ll save writing CSS.