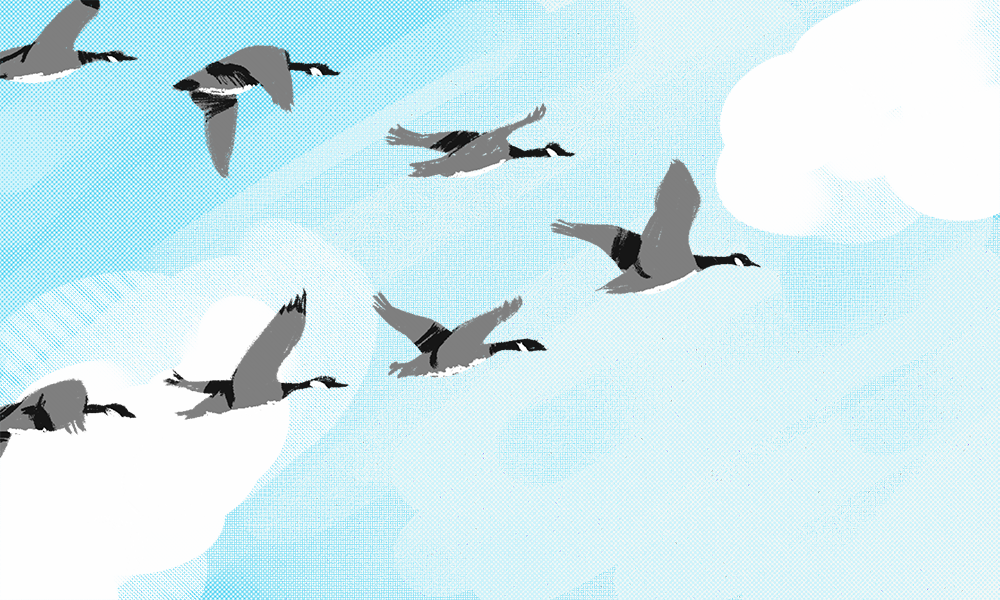
As of Fall 2018 and the release of Drupal 8.6, the Migrate module in core is finally stabilizing! Hopefully Migrate documentation will continue to solidify, but there are plenty of gaps to fill.
Recently, I ran into an issue migrating Paragraph Entities (Entity Reference Revisions) that had a few open core bugs and ended up being really simple to solve within
In your migration yml file, you can reference the
Code
Drupal
Drupal 8
Drupal Planet
JavaScript
prepareRow
in the source plugin.
Setup
In my destination D8 site, I had a content type with a Paragraph reference field. Each node contained one or more Paragraph entities. This was reflected by having a Node migration with a dependency on a Paragraph Entity migration. With single value entity references, themigration_lookup
plugin makes it really easy lookup up entity reference identifiers that were previously imported. As of September 2018, there is an open core issue to allow multiple values with migration_lookup
. Migration lookup uses the migration map table created in the database to connect previously migrated data to Drupal data. The example below lookups up the taxonomy term ID based on the source reference (topic_area_id) from a previously ran migration. Note: You will need to add a migration dependency to your migration yml file to make sure migrations are run in the correct order.
field_resource_category: plugin: migration_lookup migration: nceo_migrate_resource_category no_stub: true source: topic_area_id
Solution
Without using a Drupal Core patch, we need a way to do amigration_lookup
in a more manual way. Thankfully prepareRow
in your Migrate source plugin makes this pretty easy.
Note: This is not a complete Migrate source plugin. All the methods are there, but I’m focussing on the prepareRow method for this post. The most important part of the code is manually querying the Migrate map database table created in the Paragraph Entity migration.
<?php namespace Drupal\your_module\Plugin\migrate\source; use Drupal\Core\Database\Database; use Drupal\migrate\Plugin\migrate\source\SqlBase; use Drupal\migrate\Row; /** * Source plugin for Sample migration. * * @MigrateSource( * id = "sample" * ) */ class Sample extends SqlBase { /** * {@inheritdoc} */ public function query() { // Query source data. } /** * {@inheritdoc} */ public function fields() { // Add source fields. } /** * {@inheritdoc} */ public function getIds() { return [ 'item_id' => [ 'type' => 'integer', 'alias' => 'item_id', ], ]; } /** * {@inheritdoc} */ public function prepareRow(Row $row) { // In migrate source plugins, the migrate database is easy. // Example: $this->select('your_table'). // Getting to the Drupal 8 db requires a little more code. $drupalDb = Database::getConnection('default', 'default'); $paragraphs = []; $results = $drupalDb->select('your_migrate_map_table', 'yt') ->fields('yt', ['destid1', 'destid2']) ->condition('yt.sourceid2', $row->getSourceProperty('item_id'), '=') ->execute() ->fetchAll(); if (!empty($results)) { foreach ($results as $result) { // destid1 in the map table is the nid. // destid2 in the map table is the entity revision id. $paragraphs[] = [ 'target_id' => $result->destid1, 'target_revision_id' => $result->destid2, ]; } } // Set a source property that can be referenced in yml. // Source properties can be named however you like. $row->setSourceProperty('prepare_multiple_paragraphs', $paragraphs); return parent::prepareRow($row); } }
prepare_multiple_paragraphs
that was created in the migrate source plugin like this:
id: sample label: 'Sample' source: plugin: sample process: type: plugin: default_value default_value: your_content_type field_paragraph_field: source: prepare_multiple_paragraphs plugin: sub_process process: target_id: target_id target_revision_id: target_revision_id
Sub_process
was formally the iterator
plugin and allows you to loop over items. This will properly create references to multiple Paragraph Entities. It will be nice when the migration_lookup plugin can properly handle this use case, but it’s a good thing to understand how prepareRow can provide flexibility.
Skip to footer
Comments