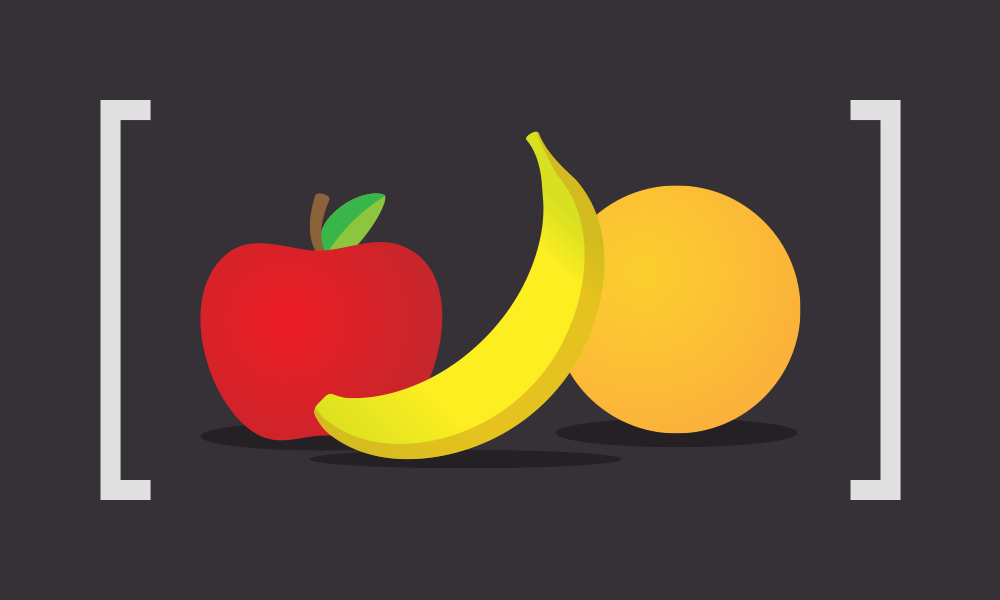
Lately, I’ve been working on an exciting data visualization project for one of our clients. This requires a lot of data manipulation as data is pulled from the API server and transformed into visualizations. I found myself using the same array methods over and over to get this work done–Map, Filter and Reduce.
Map, Filter, and Reduce are used to take an array of things and turn them into something else. Here’s how I like to look at them.
Map
You have an array of items and want to transform each of them. The result is a new array of the exact same length containing the manipulated items.
Filter
You have an array and want to filter out certain items. The result is a new array with the same items, but with some excluded. The length of the new array will be the same (if no values were omitted) or shorter than the original.
Reduce
You have an array of items and you want to compute some new value by iterating over each item. The result can be anything, another array, a new object, a boolean value etc.
Map, Filter, and Reduce do not manipulate the original array. In each case, you end up with a brand new value. Let’s look at some examples.
Array.map()
Let’s say we have an array of objects representing various Transformers (The 1980’s G1 Transformers, not that terrible Michael Bay junk.)
<br /> var transformers = [<br /> {<br /> name: 'Optimus Prime',<br /> form: 'Freightliner Truck',<br /> team: 'Autobot'<br /> },<br /> {<br /> name: 'Megatron',<br /> form: 'Gun',<br /> team: 'Decepticon'<br /> },<br /> {<br /> name: 'Bumblebee',<br /> form: 'VW Beetle',<br /> team: 'Autobot'<br /> },<br /> {<br /> name: 'Soundwave',<br /> form: 'Walkman',<br /> team: 'Decepticon'<br /> }<br /> ];<br />
We want a new array that lists all the forms that our robots take on. Array.map() makes this easy.
The basic signature looks like this:
<br /> Array.prototype.map(callback(item));<br />
In most cases, the above will do. In more complex scenarios, the full signature looks like this:
The callback is run on each item of the array. Its return value will be the new item in the resulting array. We can write this a few different ways:
As a named function:
<br /> function getForm(transformer) {<br /> return transformer.form;<br /> }<br /> var robotsInDisguise = transformers.map(getForm);<br /> /** robosInDisguise === ['Freightliner Truck', 'Gun', 'VW Beetle', 'Walkman'] */<br />
As an anonymous function:
<br /> robotsInDisguise = transformers.map(function(transformer) {<br /> return transformer.form;<br /> });<br />
As an arrow function:
<br /> robotsInDisguise = transformers.map(transformer => transformer.form);<br />
The above ES2015 arrow function syntax is super handy for quick maps where you just want to pull a simple computed value out of each item of an array.
Array.filter()
Array.filter allows us to take an array of items and filter it down to just the items we want.
The full function signature is
<br /> Array.prototype.filter(callback(item));<br />
The callback takes the item as an argument and returns a boolean value. If it returns true, the item is added to the new array. If it returns false, the item is left out.
Let’s filter our list of Transformers down to just Autobots.
<br /> function isAutobot(transformer) {<br /> return transformer.team === ‘Autobot’;<br /> }</p> <p>var autobots = transformers.filter(isAutobot);<br /> /**<br /> autobots == [<br /> {<br /> name: 'Optimus Prime',<br /> form: 'Freightliner Truck',<br /> team: 'Autobot'<br /> },<br /> {<br /> name: 'Bumblebee',<br /> form: 'VW Beetle',<br /> team: 'Autobot'<br /> }<br /> ]<br /> */<br />
Array.reduce()
Array.reduce() allows you to compute a single value by iterating over a list of items. Reduce is the trickiest of the three array methods we’ve looked at as the signature is a bit more complex.
<br /> Array.prototype.reduce(callback(previousValue, currentValue[, index], array]), initialValue)<br />
Just remember, we have an array of items and want to reduce them to a single value. Let’s say we have an array of Construction Transformers. Constructicons are construction robots that combine, Voltron style, into a giant robot called Devastator. Let’s code that for pretend.
<br /> var constructicons = [<br /> {<br /> name: 'Scrapper',<br /> form: 'Freightliner Truck',<br /> team: 'Decepticon',<br /> bodyPart: 'rightLeg'<br /> },<br /> {<br /> name: 'Hook',<br /> form: 'Mobile Crane',<br /> team: 'Decepticon',<br /> bodyPart: 'upperTorso'<br /> },<br /> {<br /> name: 'Bonecrusher',<br /> form: 'Bulldozer',<br /> team: 'Decepticon',<br /> bodyPart: 'leftArm'<br /> },<br /> {<br /> name: 'Scavenger',<br /> form: 'Excavator',<br /> team: 'Decepticon',<br /> bodyPart: 'rightArm'<br /> },<br /> {<br /> name: 'Mixmaster',<br /> form: 'Concrete Mixer',<br /> team: 'Decepticon',<br /> bodyPart: 'leftLeg'<br /> },<br /> {<br /> name: 'Long Haul',<br /> form: 'Dump Truck',<br /> team: 'Decepticon',<br /> bodyPart: 'lowerTorso'<br /> }<br /> ];<br />
The reduce callback takes at least two arguments. The first is the previous value returned from the last iteration. The second is the current value being iterated over in the array. The return value gets passed on as the 1st argument in the next iteration. The return value of the initial reduce call will be the return value from the callback on the final iteration.
<br /> function assemble(combiner, transformer) {<br /> // On each iteration, add the current transformer to the form object of the combiner.<br /> combiner.form[transformer.bodyPart] = transformer.name;<br /> return combiner;<br /> }<br />
When we call reduce on the array; the first argument is the callback; the second is the initial value passed that callback on the first iteration. In the below example begin with a new Transformer with just the name and team values set. On each iteration of assemble, we add a Constructicon to the form property, until it is fully assembled.
<br /> var devastator = constructicons.reduce(assemble, {<br /> name: ‘Devastator’,<br /> team: ‘Decepticon’,<br /> form: {}<br /> });</p> <p>/**<br /> devastator == {<br /> name: ‘Devastator’,<br /> team: ‘Decepticon’,<br /> form: {<br /> leftArm: "Bonecrusher"<br /> leftLeg: "Mixmaster"<br /> lowerTorso: "Long Haul"<br /> rightArm: "Scavenger"<br /> rightLeg: "Scrapper"<br /> upperTorso: "Hook"<br /> }<br /> }<br /> */<br />
These basic methods can be super powerful when combined together. This is just small sample of the methods I find myself using often. Checkout MDN's great documentation for more!