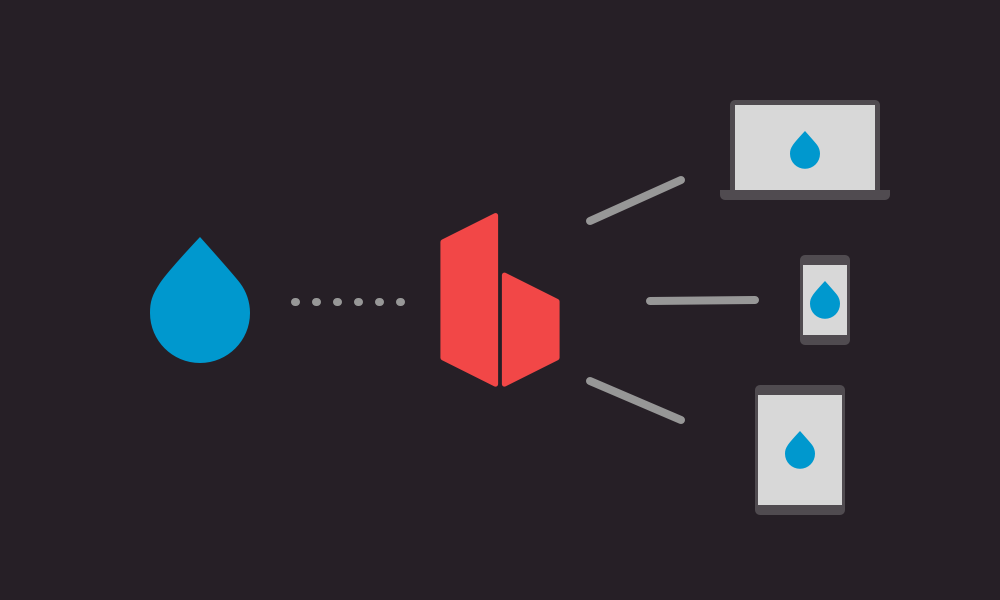
TL;DR: Just here for Browsersync setup? Skip to the steps.
I’m always looking for ways to reduce the time between saving a file and seeing changes on the screen.
When I first started working with Drupal, back in the day, I would ftp changes to a server and refresh the page. Once I found Transmit, I could mount a remote directory locally and automatically save files to the server. At the time, my mind was blown by the efficiency of it. In hindsight, it seems so archaic.
Then I started working with development teams. Servers were typically managed with version control. If I wanted to make changes to the server, I had to commit code and push it up. It was time to act like a grown up and develop with a local server.
The benefits of developing locally are too numerous to list here, but the most obvious is not having to push changes to a remote server to see the effects. I could just save a file, switch to the browser and refresh! What was taking minutes was reduced to seconds.
My progression of development practices went something like this.
“Oy, hitting cmd+r is so tedious. What’s this? LiveReload? OH MY GOD! It refreshes the page immediately upon saving! It even injects my CSS without refreshing the page! I’m so super fast right now! Whoa! Less! I can nest all my CSS so it matches the DOM exactly! Well that was a terrible idea. Not Less’s fault but ‘Hello Sass!’. Because Compass! And Vertical Rhythm! And Breakpoint! And Susy! Holy crap, it’s taking 8-12 seconds to compile CSS? Node sass you say?. That’s so much faster! Back down to 3 seconds. Wait a minute, everything I’m using Compass for, PostCSS handles. BOOM! Milliseconds!”
This boom and bust cycle of response times of mine has been going on for years and will continue indefinitely. Being able to promptly see the effects of your changes is incredibly important.
In 1968, Robert Miller published a paper in which he defined 3 thresholds of human-computer response times.
- .1 sec. To feel like you are directly manipulating the machine
- .1 - 1 sec. To feel like you are uninhibited by the machine, but long enough to notice a delay
-
10 sec. At this point, the user has lost focus and is likely to move onto another task while waiting for the computer.
In short, less than a second between making a file change and seeing the result is ideal. More than that and you’re likely to lose focus and check your email, Slack or Twitter. If it takes longer than 10 sec. to compile your code and refresh your browser, your productivity is screwed.
One of my favorite tools for getting super fast response times is Browsersync. Browsersync is a Node.js application that watches your files for changes then refreshes your browser window or injects new CSS into the page. It’s similar to LiveReload but much more powerful. The big difference is that Browsersync can be run in multiple browsers and on multiple devices on the same LAN at the same time. Each connected browser will automatically refresh when code changes. It also syncs events across browsers. So if you scroll the page in one browser, all your connected browsers scroll. If you click in one browser, all browsers will load the new page. It’s incredibly useful when testing sites across devices.
For simple sites, Browsersync will boot up its own local http server. For more complex applications requiring their own servers, such as Drupal, Browsersync will work as a proxy server. Setup is pretty simple but I’ve run into a few gotchas when using Browsersync with Drupal.
Setting up Browsersync
Local dev environment
First things first, you’ll need a local dev environment. That’s beyond the scope of this post. There are a few great options out there including DrupalVM, Kalabox and Acquia Dev Desktop.
For this tutorial, we’ll assume your local site is being served at http://local.dev
Install Node
Again, out of scope. If you don’t already have node installed, download it and follow the instructions.
Install Browsersync
Browsersync needs to be installed globally.
<br /> npm install browser-sync -g<br />
Change directories to your local site files.
<br /> cd wherever/you/keep/your/drupal/docroot<br />
Now you can start Browsersync like so:
<br /> browser-sync start --proxy "local.dev" --files "**/<em>.twig, **/</em>.css, **/*.js”<br />
The above will start a browsersync server available at the default http://localhost:7000. It will watch for changes to any Twig templates, CSS or JS files. When a change is encountered, it will refresh any browsers with localhost:7000 open. In the case of CSS, it will inject just the changed CSS file into the page and rerender the page rather than reloading it.
Making Drupal and Browsersync play nicely together
There’s a few tweaks we’ll need to do to our Drupal site to take full advantage of our Browsersync setup.
Disable Caching
First we want to make sure page caching is disabled so when we refresh the page, it’s not serving the old cached version.
Follow the steps outlined under “Enable local development settings” in the Disable Drupal 8 caching documentation.
Now when you change a Twig template file, the changes will be reflected on the page without having to rebuild the cache. Keep in mind, if you add a new file, you’ll still need to rebuild the cache.
Avoid loading CSS with @import statements
This gotcha was much less obvious to me. Browsersync will only recognize and refresh CSS files that are loaded with a link tag like so:
<br /> <link rel="stylesheet" href="/sites/.../style.css" media="all"><br />
It does not know what to do with files loaded via @import tags like this:
<br /> <style><br /> @import url('/sites/.../style.css');<br /> </style><br />
This is all well and good as Drupal uses link tags to import stylesheets unless you have a whole lot of stylesheets, more than 31 to be exact. You see, IE 6 & 7 had a hard limit of 31 individual stylesheets. It would ignore any CSS files beyond that. It’s fairly easy to exceed that maximum when CSS aggregation is turned off as any module or base theme installed on your site can potentially add stylesheets. Drupal has a nice workaround for this by switching to the aforementioned @import statements if it detects more than 31 stylesheets.
We have two ways around this.
1. Turn preprocessing off on specific files
The first involves turning CSS aggregation (a.k.a. CSS preprocessing) on for your local site and manually disabling it for the files you are actually working with.
In settings.local.php, set the following to TRUE
:
<br /> $config['system.performance']['css']['preprocess'] = TRUE;<br />
Then in your [theme_name].libraries.yml file, turn preprocessing off for any files you are currently working on, like in the following example.
<br /> global:<br /> version: 1.0.x<br /> css:<br /> theme:<br /> build/libraries/global/global.css: { preprocess: false }<br />
This will exclude this file from the aggregated CSS files. This approach ultimately leads to a faster site as your browser loads considerably fewer CSS files. However, it does require more diligence on your part to manage which files are preprocessed. Keep in mind, if you commit the above libraries.yml file as is, the global.css file will not be aggregated on your production environment as well.
2. Use the Link CSS module
The easier alternative is to use the Link CSS module. When enabled, this module will override Drupal’s @import workaround and load all files via the link tag regardless of how many. The only downside to this approach is the potential performance hit of still loading all the unaggregated CSS files which may not be a big deal for your environment.
Add a reload delay if needed
In some cases, you may want to add a delay between when Browsersync detects a change and when it attempts to reload the page. This is as simple as passing a flag to your browsersync command like so.
<br /> browser-sync start --proxy "d8.kbox.site" --files "**/<em>.twig, **/</em>.css, **/*.js" --reload-delay 1000<br />
The above will wait 1 second after it detects a file change before reloading the page. The only time I’ve ever needed this is when using Kalabox as a local development environment. Your mileage may vary.
In addition to the reload delay flag, Browsersync has a number of other command line options you may find useful. It’s also worth noting that, if the command line approach doesn’t suit you, Browsersync has an api that you can tie into your Gulp or Grunt tasks. Here at Aten we use Browsersync tied into gulp tasks so a server can be started up by passing a
serve
flag to our build task. Similar to the following gulp compile --watch --serve