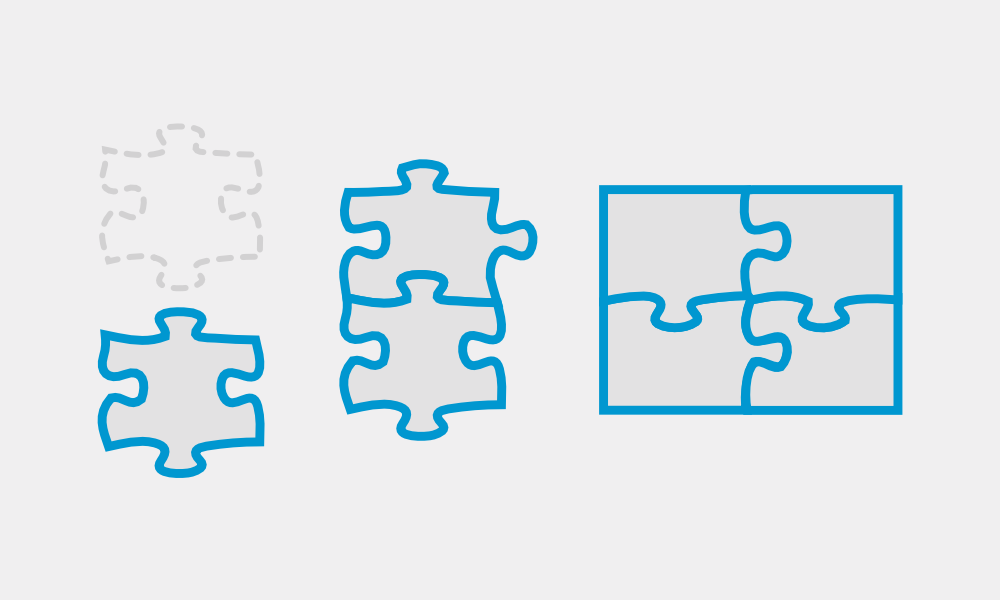
"If you're not testing, you're doing it wrong." I can't remember how many times I've heard those words. Each time, I'd feel a little pang of guilt, a little bit of shame that every day, I wrote code for myself and clients that wasn't tested. I'd be frustrated with the developers who repeated that mantra. Sure, it was easy to say, but hard to live up to. How do I test? What do I test? Should I test? How would I justify the costs? As a developer who started his career writing custom code for Drupal applications, it was easy to skip testing. Drupal 7 was really quite untestable, at least in any practical sense. Drupal core itself was tested, and big modules like Views, Ctools, and Entity API had test suites too. But finding tests in other custom code or smaller modules was a rarity. Even today, with a vastly more testable code base, documentation for testing Drupal code is terse and hard to come by. It's focused on educating core contributors and module maintainers, not day-to-day developers writing modules to satisfy particular real-world needs. I hope this series will change that for you. I will make the case that you should be testing; that you'll save time and you'll save money, and that you won't need to "justify the cost." This series will start from first principles: * What is automated testing? * Why is automated testing a net positive? * How do I write my first test? * How do I write the one after that? (because that's really where it gets hard isn't it?) ## Part Zero ### What is automated testing? I define automated testing as the act of asserting that your code achieves its goals without human interaction. ### Types of Automated Tests There are many types of automated testing. Each serves a specific need and each operates at a different level of abstraction. They form something like a pyramid, your most numerous tests should be at the bottom, as you go higher up the stack, you should have fewer and fewer tests.
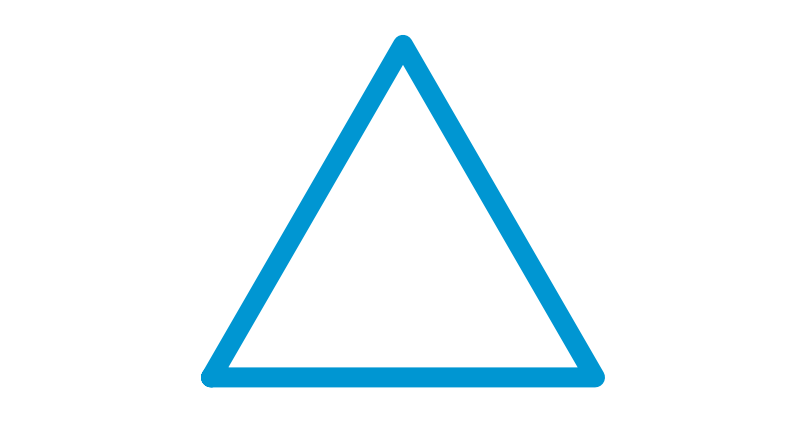
At the lowest level, are **unit tests** (and that's what this series will focus on). Unit testing is code that you write to test or "exercise" the actual custom code that you write. Unit tests isolate very small bits of functionality and assert that your code can handle all kinds of inputs correctly. You might write a unit test to assert that an add(x, y) function adds numbers correctly.
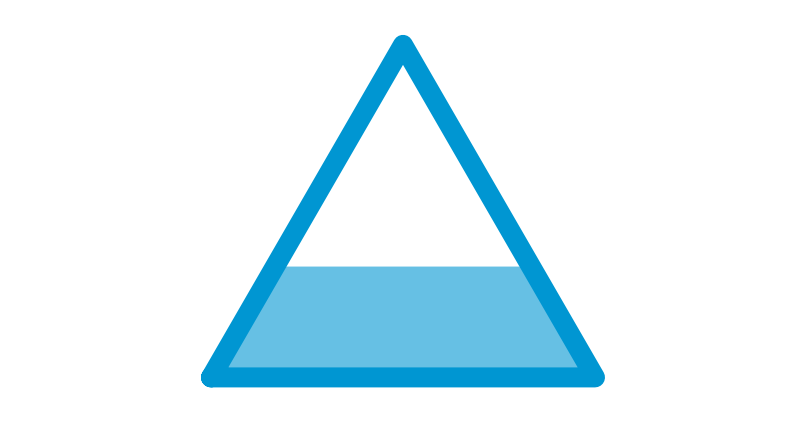
Above unit tests, are **integration tests**. Integration tests assert that the small bits of functionality that you tested with unit tests "integrate" together. Perhaps you wrote a suite of arithmetic functions which you unit tested individually. When those functions come together into a calculator, you might write integration tests to validate that they all work in harmony.
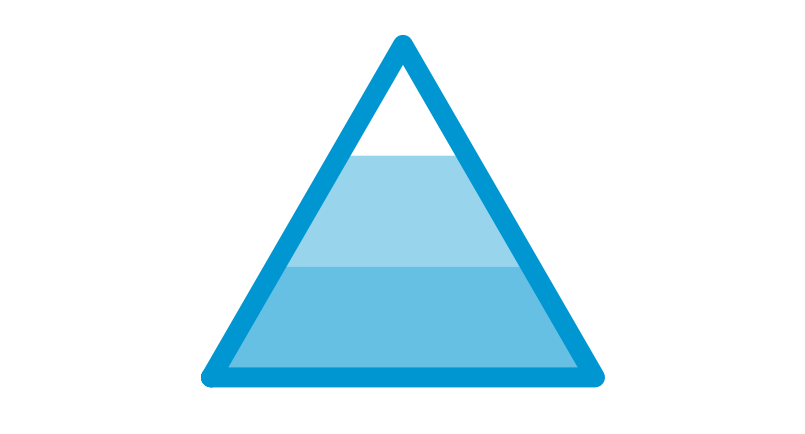
At the highest level are **system tests**. System tests assert that your application works as a cohesive whole. These "acceptance" tests are usually best expressed as the functionality your client cares about. "Can my potential customer use a calculator to estimate a mortgage payment and then call me for a quote?"
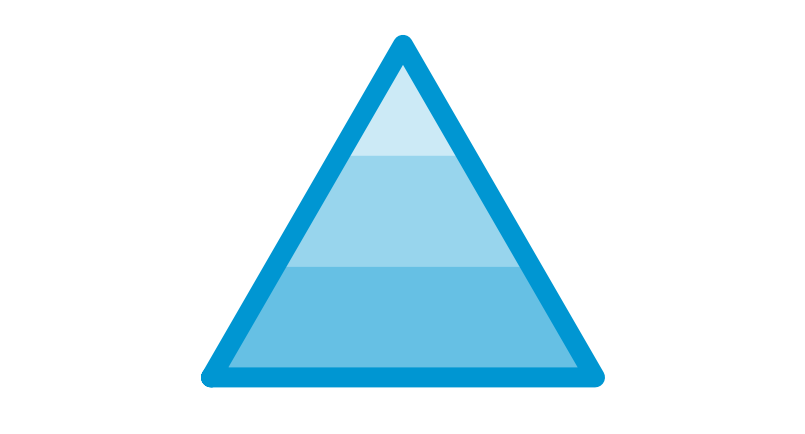
There are no definite lines of separation between these types of tests, they all fall along a continuum—it's a curve, not a step function. It's not important to know exactly where your test falls on that curve, really, it's just important to know that: * You can test at different levels of abstraction. * You do not need to test *everything* at every level of abstraction. ### Different Tools for Different Tests Just as there are different types of tests, there are different tools that go along with them. As with all things in software development, there are lots of tooling choices and tradeoffs no matter what you choose. The beauty of using Drupal, however (or any framework) is that some of those choices have already been made for you either officially or by convention in the community. At the lowest level is **unit testing**. The standard adopted by Drupal 8 is PHPUnit. PHPUnit is a suite of command line tools and base classes that make writing tests easier. Drupal has extended some of the PHPUnit classes with some extra features that make testing code written specifically for Drupal easier. The Drupal class used for unit testing is called [UnitTestCase](https://api.drupal.org/api/drupal/core!tests!Drupal!Tests!UnitTestCase.php/class/UnitTestCase/8.2.x). We're going to take a deep dive into this, and all the Drupal testing classes and tools later in the series. At the **integration test** level, Drupal uses a mix of PHPUnit and Simpletest, but is migrating all of its Simpletest based code to extensions of PHPUnit tests that can achieve the same things. In Drupal, the class primarily used for this kind of testing is called [KernelTestBase](https://api.drupal.org/api/drupal/core%21tests%21Drupal%21KernelTests%21KernelTestBase.php/class/KernelTestBase/8.2.x). At the **system test** level the lines begin to become somewhat blurred. Drupal calls these “Functional” tests and there are two classes for them. [WebTestCase](https://api.drupal.org/api/drupal/core%21modules%21simpletest%21src%21WebTestBase.php/class/WebTestBase/8.2.x) and [BrowserTestBase](https://api.drupal.org/api/drupal/core%21tests%21Drupal%21Tests%21BrowserTestBase.php/class/BrowserTestBase/8.2.x) classes can do quite a bit, and are the standard for testing Drupal Core and contributed modules. They work well for contributed modules and Drupal core because they don’t need to test the specifics of a real-world Drupal application and all the configuration and customization that implies.
The Drupal community has largely settled on Behat as the standard for testing real-world Drupal applications. Behat works by starting a "headless" browser that can emulate a real user by doing things like clicking links and filling out forms. These kinds of tests let you test the configuration of your Drupal site holistically—your theme, javascript and custom code—which ensures that everything works well together. I hope this post has given you a sense of what automated testing is and some basic terminology that we can share in the next part of this series: “Why Automated Testing will Save You Time and Treasure.”