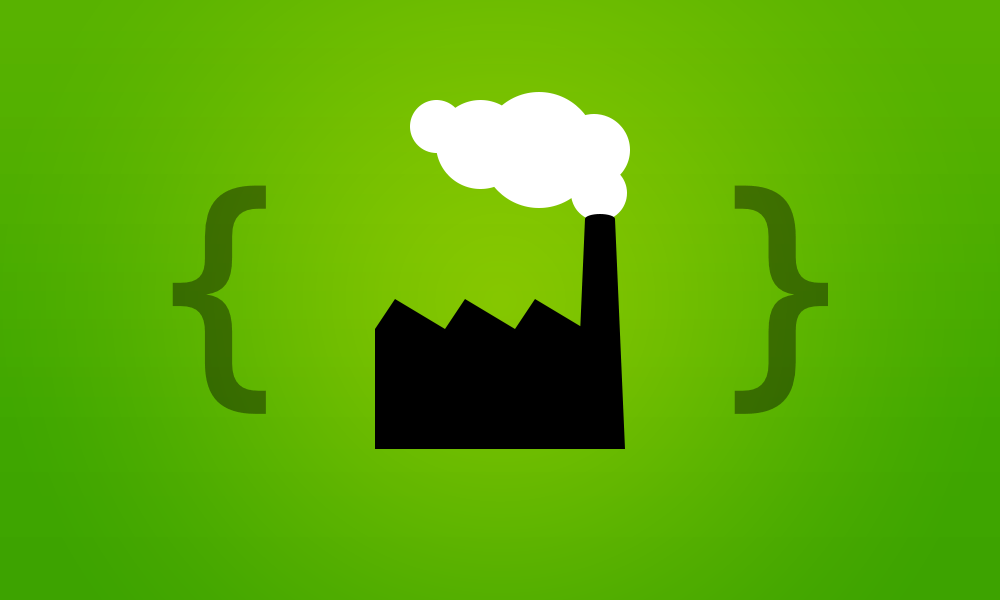
As we move from an age of jQuery plugins and script drop-ins to a world of CommonJS and modular architectures it's becoming increasingly more important to manage JavaScript wisely. One of the features currently missing from JavaScript is the ability to create classes, although that will soon change with ES6. There are multiple methods and patterns to try and recreate this functionality. One of those patterns is Factory Functions.
When you need to add functionality to an existing object, factory functions allow you to add it with ease and very little refactoring. Take a look at the code example below of a factory function.
<br /> // Function factory<br /> function Car () {<br /> var self = {<br /> make: 'Honda',<br /> model: 'Accord',<br /> color: '#cc0000',<br /> paint: function(color){<br /> self.color = color;<br /> }<br /> };<br /> return self;<br /> }</p> <p>var myCar = Car();<br />
As you can see, factory functions aren't all that different from other methods of building modular JavaScript.
Simple Syntax
Not only will using factory functions help you in the long run but they are also very simple to code initially. When creating a constructor function you must use 'new' to make sure it instantiates a new object. 'new' allows us to be able to use the 'this' variable inside of our constructor. 'this' allows us to apply properties, methods, and events to our new instantiated object. Using the constructor method is also very rigid in terms of structure. You have to follow the rules in order to properly return and instantiate an object.
However, with factory functions the syntax is much simpler. No need to call ‘new’ in front of your constructor, you can simply call the function and attach it to a variable:
<br /> var myCar = Car();<br />
You also have more access to the 'this' variable in factory functions. In factory functions ‘this’ refers to the parent object, so in the function myCar.paint(), ‘this’ refers to ‘myCar’. While in constructor functions ‘this’ refers to the method and not the parent object.
Hello Private/Public functions
When using the function factory architecture you have flexibility in how you build your object. Factory functions allow you to define private variables and methods inside of the instantiation function. In the example below, the ‘Car’ function has a private method of year() and a private variable of location. Both this method and the variable are not accessible from outside the car function.
<br /> function Car () {<br /> // private variable<br /> var location = 'Denver';<br /> function year() {<br /> self.year = new Date().getFullYear();<br /> }</p> <p> var self = {<br /> make: 'Honda',<br /> model: 'Accord',<br /> color: '#cc0000',<br /> paint: function(color){<br /> self.color = color;<br /> }<br /> };</p> <p> if (!self.year){<br /> year();<br /> }</p> <p> return self;<br /> }</p> <p>var myCar = Car();<br />
Since the year method is private, the following will NOT work:
<br /> var myCar = Car();<br /> myCar.year();<br />
Your console will show an error of an undefined function. If you wanted to run the year() function outside of the initial ‘Car’ initialization you would have to add the year() method in the self object. Like this:
<br /> function Car () {<br /> var self = {<br /> make: 'Honda',<br /> model: 'Accord',<br /> color: '#cc0000',<br /> paint: function(color){<br /> self.color = color;<br /> },<br /> year: function(){<br /> return self.year = new Date().getFullYear();<br /> }<br /> };</p> <p> return self;<br /> }</p> <p>var myCar = Car();<br /> myCar.year();<br />
Dynamic Objects
This also brings up the fact that factory functions are very dynamic. Since we can have public/private functions we can use if/else statements to easily manipulate our object structure. This gives ultimate flexibility to allow the root function ambiguity and allow parameters to determine what the object returned should be.
For example, if you wanted to add specific development methods to your object based on an environment variable you could easily pass the factory function a parameter and adjust the object accordingly.
<br /> function Address (param) {<br /> var self = {};</p> <p> if (param === 'dev'){<br /> self = {<br /> state: ‘Colorado’,<br /> saveToLog: function(){<br /> // write info to a log file<br /> }<br /> };<br /> } else {<br /> self = {<br /> state: 'Colorado'<br /> };<br /> }</p> <p> return self;<br /> }</p> <p>var devAddress = Address('dev');<br /> var productionAddress = Address();<br />
You can see in the example above that we can allow the object to fit the needs of parameters. In our development environment we add a method that can save information to a log file, whereas, our production version can be without this method and also be lighter in memory. This gives us a more granular way of controlling our object.
Overall, factory functions are very future-proof, flexible and dynamic. Using them to write our JavaScript will allow for a more controlled architecture that can be easily maintained throughout the life of a project.