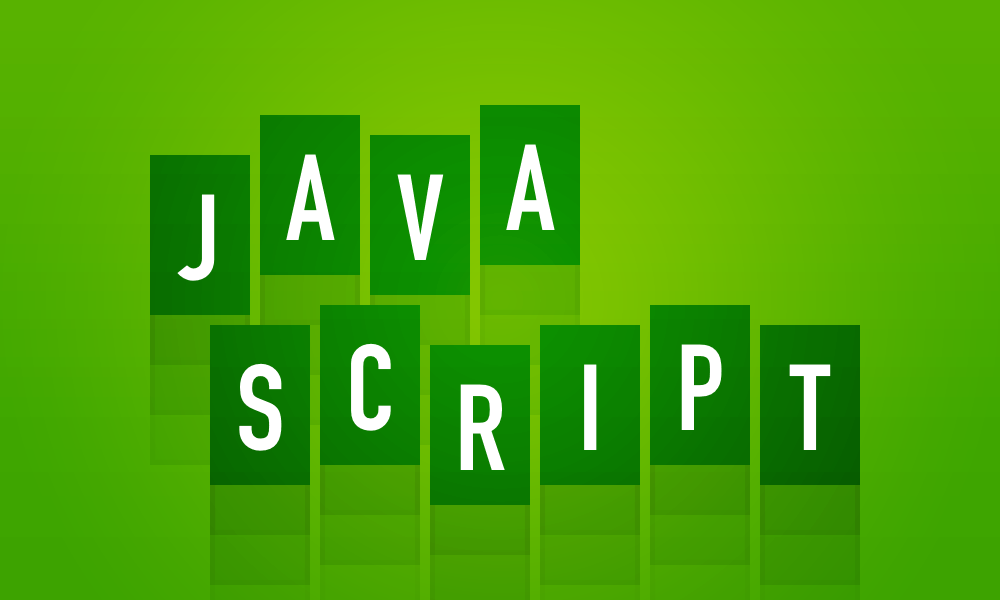
I came to Drupal from a JavaScript background and was happy to see a module
system in place. Have you ever wished you could write your JavaScript modularly
just like your PHP in order to take advantage of testing, better documentation
and the Do One Thing Well philosophy? Well I have the
solution for you: Browserify and NPM!
Getting Started
In order to take advantage of JavaScript modularity you will need
node
and npm
which can be installed together using
one of these methods.
Creating a JavaScript Module
JavaScript modules are similar to the PHP include system in that they allow
the developer to separate their code across multiple files. The key difference
is that JavaScript uses the syntax require
to pull the exported
part of a JavaScript file into the current file's variable of choice. Let's take a look.
Simply create a JavaScript file, do whatever you’re trying to do and assign
the resulting value (usually a class or object) to module.exports
.
module.exports = function MyClass(opts) {}
MyClass.prototype.magic = function() {
console.log(‘magic’);
}
You can now use MyClass.js
from other JavaScript files like so:
var classy = new MyClass();
classy.magic(); // ‘magic’
NPM
PHP developers may be familiar with Composer. NPM is the Composer
of the JavaScript world. Both allow you to manage the dependencies of
your project.
Before creating a custom module it's a great idea to see if it has already been
written. npmjs.org is the place to look. When you find a module you’d
like to include in your project, retrieve it and add it as a dependency with
npm
. For example, to install lodash:
<br /> npm install lodash --save<br />
The save flag adds this dependency to the
package.json
file.It might help to think of this as the JavaScript version of
composer.json.
All the dependencies for a project can be installed by running
npm install
withinthe directory containing
package.json
or its sub-directories.Requiring modules from NPM is done just like local modules but you don’t
have to provide the path, just use the module name.
require
will look inthe
node_modules
folder automatically.
Read more about NPM and package.json on the NPM website.
The Browserify command
Browserify reads your JavaScript files and resolves their module.exports
and require
's.
Your main JavaScript file should be a compilation of its dependencies
resulting in a useful piece of functionality for your website. To compile your
multiple files into one that is browser ready we will use the
browserify CLI.
First install it globally with npm
. Globally installed modules can be accessed
from anywhere on your machine.
<br /> npm install -g browserify<br />
Then tell Browserify where your main JavaScript file is and redirect the output
to where you want your compiled, browser-ready JS to be.
<br /> browserify src/index.js > build/myModule.js<br />
build/myModule.js
is now browser ready! Now you can add it to your website.
Come see me at BADCamp
The above workflow is a great way to maintain custom Drupal modules with a lot of
JavaScript. For more detailed information about using this workflow with Drupal —
such as global dependencies, handling jQuery, task automation and testing — come check out my session at this year’s BADCamp!