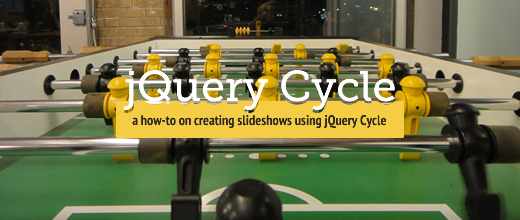
When it comes to web development, it would hurt my feelings if you called me a one-trick-pony. However, for the majority of projects I've worked on, there has been a need for a slideshow and/or rotating content. For a number of years, SlideShowPro (a flexible Flash slideshow), was my goto for slideshows. SlideShowPro was great, and had the flexibility to include video, but customizing the look of the slideshow and outputting correctly formatted XML for it to consume was a pain. In recent years, I've moved away from Flash based slideshow solutions in favor of JavaScript solutions.
If you've been doing web development for a while, you've likely heard of jQuery Cycle. jQuery Cycle is a jQuery plugin that allows developers to easily create flexible slideshows. The jQuery Cycle site has plenty of good examples and documentation, so I won't spend too much time on code. In it's most basic form, the slideshow takes very little code (be sure to view the source).
Why I love It
1. Rotate just about anything
jQuery Cycle will rotate most types of content, not just images. You just need a parent html element, and it will rotate the children elements.
Example Markup One
<br /> <pre><br /> <ul id="slideshow"><br /> <li class="slide"><img src="http://placehold.it/350x150" alt="Image 1" /></li><br /> <li class="slide"><img src="http://placehold.it/350x150" alt="Image 2" /></li><br /> <li class="slide"><img src="http://placehold.it/350x150" alt="Image 3" /></li><br /> </ul><br /> </pre><br />
Example Markup Two
<br /> <pre><br /> <div id="slideshow"><br /> <div class="slide"><br /> <img src="http://placehold.it/350x150" alt="Image 1" /><br /> <p>Additional Content</p><br /> </div><br /> <div class="slide"><br /> <img src="http://placehold.it/350x150" alt="Image 2" /><br /> <p>Additional Content</p><br /> </div><br /> <div class="slide"><br /> <img src="http://placehold.it/350x150" alt="Image 3" /><br /> <p>Additional Content</p><br /> </div><br /> </div><br /> </pre><br />
2. Plenty of Options
jQuery Cycle has lots of options that make it really flexible. Some of my favorites include:
- before: Allows you to call a function that will happen before the slideshow transitions.
- after: Allows you to call a function that will happen after the slideshow transitions. Useful for fading in a caption or updating a count (x of y slides)
- pagerAnchorBuilder: Allows you to call a function to build slideshow navigation with specific markup
3. Ease of Control a.k.a. Slideshow Navigation
jQuery cycle makes using custom play, pause, previous/next controls simple.
Markup needed
<br /> <pre><br /> <ul id="slideshow-controls"><br /> <li><a href="#" id="previous">Previous</a></li><br /> <li><a href="#" id="play-pause">Pause</a></li><br /> <li><a href="#" id="next">Next</a></li><br /> </ul><br /> </pre><br />
Note: We typically insert the navigation markup via JavaScript so if a user has JavaScript disabled, they aren't left with a broken slideshow. Also, you can add the css property "overflow: hidden" to the slideshow container, and it will only show the first item in your slideshow if JavaScript is disabled.
jQuery needed
<br /> <pre><br /> //Initiate jQuery Cycle<br /> $('#slideshow').cycle({<br /> prev: '#previous', //#id of your previous button<br /> next: '#next' //#id of your next button<br /> });</p> <p>//Handle pausing and playing<br /> $('#play-pause').click(function() {</p> <p> //Check to see if the slideshow is currently paused.<br /> if ($(this).hasClass('paused')) {</p> <p> //Resume the slideshow, remove the class from the #play-pause button and change the text to Pause<br /> $('#slideshow').cycle('resume');<br /> $(this).removeClass('paused').text('Pause');</p> <p> } //if<br /> else {</p> <p> //Pause the slideshow, add the paused class to the #play-pause button and change the text to Play<br /> $('#slideshow').cycle('pause');<br /> $(this).addClass('paused').text('Play');</p> <p> } //else</p> <p> //Make sure nothing else happens when you click the #play-pause button<br /> return false;<br /> });<br /> </pre><br />
Examples
Check out a few examples where we have implemented jQuery Cycle in Aten projects.
- Aten Design Group - Rotating homepage callout with numbered navigation
- Zimmerman Marine - Rotating slideshow with custom controls
- NewsU - Rotating homepage callout with simple next and previous navigation
- Public Campaign - Rotating homepage callout with numbered navigation (usually rotates text-only content)
What are your favorite solutions for implementing slideshows and rotating content?