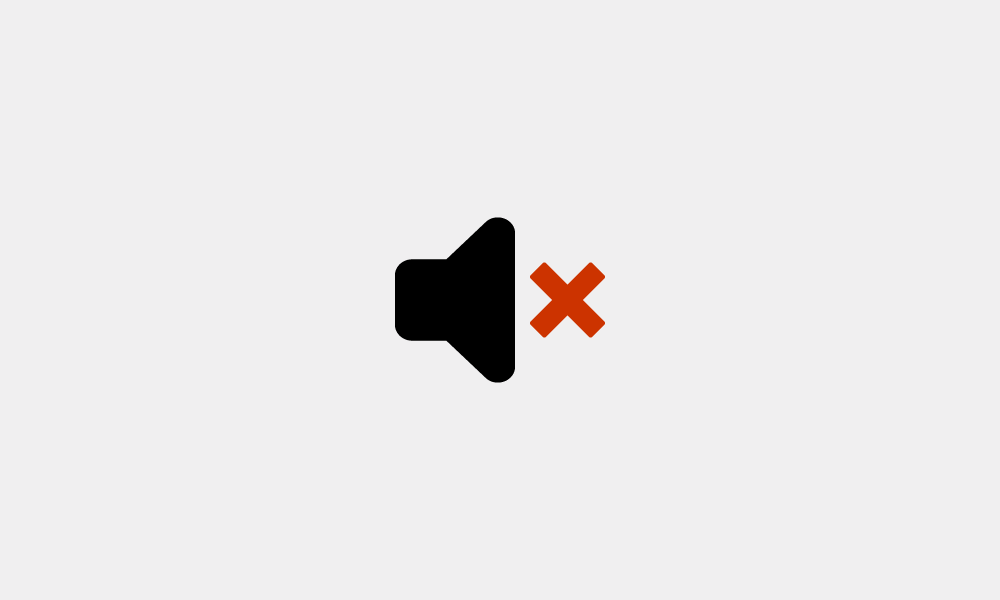
Sometimes you don’t want to show Drupal’s node creation message to your users. Or maybe you’d just like to customize a default message. You might be creating nodes for backend administrative tasks, or you might want your node creation messages to have a little more pizazz than the standard A new node of node type has been created.
Either way, you want to change or remove the node creation message. There are a couple ways to take care of it, but for the sake of copy & paste-ability I’ll go with a blanket solution. In this example, we’ll get rid of the node creation messages in two steps. I’ll touch on customizations towards the end of this post. In both cases, though, we’ll first define a custom form submission handler and then we’ll attach that handler to all of our node forms.
Define a custom form submission handler
Notification messages live in the $_SESSION variable, so our custom form submission handler will inspect the $_SESSION variable and unset the messages we don’t want. We’ll identify the unwanted node creation messages with string position matching. You could also use regular expressions if you need more flexibility. The custom handler works the same whether you’re using Drupal 7 or Drupal 8, but the function declaration is a little different.
<br /> // function mymodule_message_grooming_handler(&$form, \Drupal\Core\Form\FormStateInterface $form_state) { // Drupal 8<br /> function mymodule_message_grooming_handler(&$form, &$form_state) { // Drupal 7</p> <p> // Define an array of the messages you want to remove using their first few words<br /> $message_removal = array(<br /> "Basic page",<br /> "Article",<br /> "My content type"<br /> );</p> <p> // Remove all of the messages that match your conditions<br /> if (isset($_SESSION['messages'])) {<br /> foreach ($_SESSION['messages'] as $status => $message_array) {<br /> foreach ($_SESSION['messages'][$status] as $key => $value) {<br /> foreach ($message_removal as $message) {<br /> if (strpos($value, $message) === 0) {<br /> unset($_SESSION['messages'][$status][$key]);<br /> }<br /> }<br /> }</p> <p> // Clean up the $_SESSION array to prevent empty messages from printing to screen<br /> if (empty($_SESSION['messages'][$status])) {<br /> unset($_SESSION['messages'][$status]);<br /> }<br /> }<br /> }</p> <p>}<br />
Attach the custom form submission handler to node forms
Next we want to attach the submission handler to node forms. For this example I’m attaching the handler to every node form for ease of use. If you’d rather specify exactly which forms get the handler attached you could do that with hook_form_FORM_ID_alter(). This code will change a little depending on whether you’re using Drupal 7 or Drupal 8. We’ll do the Drupal 7 example first.
<br /> // Drupal 7<br /> function mymodule_form_alter(&$form, &$form_state, $form_id) {</p> <p> // Attach our custom submit handler to any node form<br /> if (substr($form_id, -9) == "node_form") {<br /> $form['actions']['submit']['#submit'][] = “mymodule_message_grooming_handler”;<br /> }</p> <p>}</p> <p>// Drupal 8 (we’re targeting node forms in the hook)<br /> function mymodule_form_node_form_alter(&$form, \Drupal\Core\Form\FormStateInterface $form_state) {<br /> foreach (array_keys($form['actions']) as $action) {<br /> if ($action != 'preview' && isset($form['actions'][$action]['#type']) && $form['actions'][$action]['#type'] === 'submit') {<br /> $form['actions'][$action]['#submit'][] = 'mymodule_message_grooming_handler';<br /> }<br /> }<br /> }<br />
That’s it. Make sure your module is enabled, clear your cache and start appreciating your lack of node creation messages. If you’d like to add your own custom messages you could do that in your hook_form_alter() with a drupal_set_message(), your custom submit handler by directly editing the values in the $_SESSION variable, or even with Rules if you’d rather click your way to a solution.