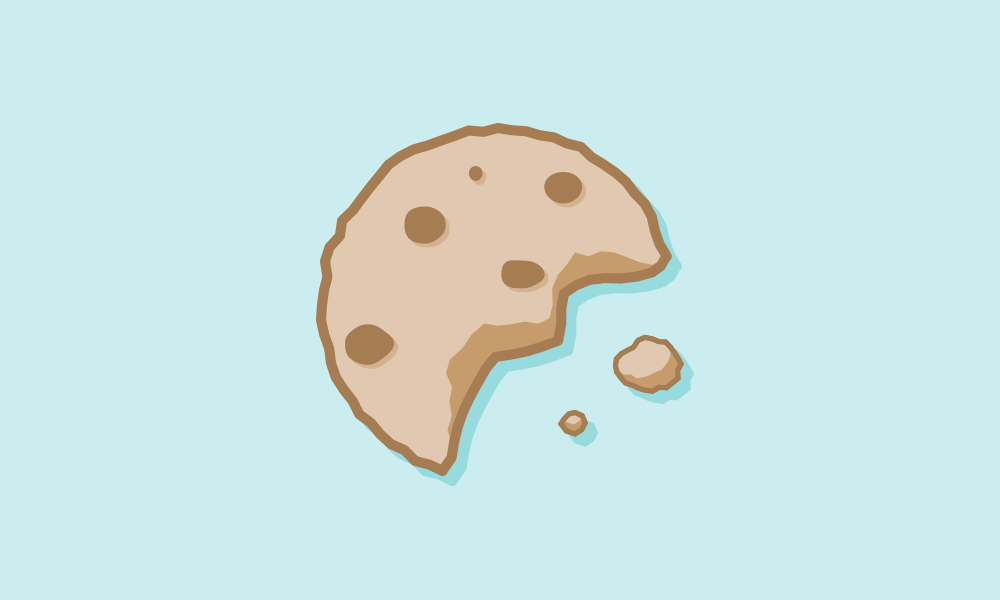
HTML5 has brought us several new technologies that make our lives as front-end developers easier. One technology that stands out among the rest is the localStorage spec. It is an easy-to-use, lightweight alternative to cookies and is perfect for storing and accessing local data quickly.
When it comes to storing user-specific data, you have backend solutions and front-end solutions. MySQL or MongoDB can store user data on a server, but you would most likely need that user to have a login to attach specific information to him or her. With front-end solutions, such as cookies or localStorage, you can tie a user’s data to their session or browser with ease.
Here at Aten I recently utilized localStorage when I built a splash page for New Climate Economy’s new climate report. In this model I used local storage to check the last time a user visited the site. If that specific user hadn’t been to the site within one week the splash page was presented; otherwise they skip the splash page and go straight to the site. Instead of using the traditional cookie-based method to check the last time a user visited the site we used local storage, which streamlines the user experience.
So why is localStorage better than using a cookie?
Give a mouse a cookie
Cookies have several drawbacks, the most important one being that they increase your page load time. Since the cookies are stored as text files they must be evaluated at each HTTP request, which can delay page loading on the user’s end. Plus 4kb is the cookie storage limit, a major obstacle when trying to promote consistent user experience. For example, if you wanted to preload a page for a user you could easily store it in localStorage without having to worry about the 4kb limit.
Oh thank heaven, HTML 5
Local storage came onto the scene as part of HTML5 and provides a much faster and simpler way to store the same type of data as cookies. Local storage is essentially its own Javascript object that is persistent in the user’s browser. As a developer, you can easily write Javascript strings or JSON objects to be stored in the localStorage object. You can see the full localStorage specification at the W3C site.
Here is an example of how to use localStorage on your site.
localStorage.setItem('splash', 'page_visited');
In the above example we have saved a simple string into local storage. Thinking back to our splash page example, a good way to show a splash page without being annoying is by setting a time limit in our local storage tracker.
var d = new Date().getTime(); localStorage.setItem('splash', d);
As you can see above, we have the date that the user visited the site saved into local storage. Now we need to set up a way of checking to see how long it has been since the user last visited.
// jQuery is loaded earlier in the page to select elements var $splash = $('.splash-page');
function hideSplash(e){ $splash.hide(); var d = new Date().getTime(); localStorage.setItem('splash', d); } $(document).ready(function(e){ var d = new Date().getTime(); // If the user has not visited in a week show splash page if (d - localStorage.getItem('splash') > 604800000){ $splash.show(); // Sets amount of time before splash is closed automatically setTimeout(hideSplash, 40000); } });
How can I save larger chunks of data?
By default local storage just saves key pair string values, so how can you save an object? If you were to save an object directly to local storage it would try to save it as a string. Luckily, JSON.stringify() can put your object into a JSON readable format.
To pull that variable back into an object we can simply use JSON.parse()
By using JSON.parse() we get our javascript object "article" back as an object and not a string.
Using local storage, you can create a modern and speedy user experience that responds to a user’s behavior and history without slowing your site down. While you can use a backend solution like MySQL and ajax, you still wouldn't be able to get the speed of localStorage. In the future this could become an even more important part of web development, as it can make websites feel more instant and app-like.