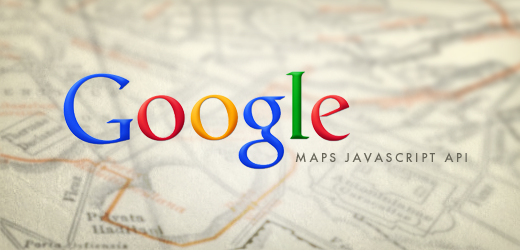
The Google Maps JavaScript API is a powerful tool for mapping solutions. With a minimal amount of code you can map points and directional routes in a clean visual way. We recently used the API to implement a solution that maps an itinerary of things to do in Southwest Virginia. We used the Drupal Services module to save itineraries. Let's take a look at the code to see how easy it is to start using the JavaScript API.
The Right Includes
The first thing you need to do is include the main JS file from Google:
<br /> <script type="text/javascript"<br /> src="http://maps.google.com/maps/api/js?sensor=false"><br /> </script><br />
This should give you access to both the mapping API and the directions API.
Initialize the Map
Next setup the map object in your JavaScript code.
</p> <p> var directionDisplay;<br /> var directionsService = new google.maps.DirectionsService();<br /> var map;</p> <p>function initializeMap() {<br /> directionsDisplay = new google.maps.DirectionsRenderer();<br /> var virginia = new google.maps.LatLng(38.23818, -80.81543);<br /> var myOptions = {<br /> zoom: 6,<br /> mapTypeId: google.maps.MapTypeId.ROADMAP,<br /> scrollwheel: false,<br /> center: virginia<br /> }<br /> map = new google.maps.Map(<br /> document.getElementById("map-canvas"), myOptions);<br /> directionsDisplay.setMap(map);</p> <p> //go ahead and calculate route<br /> plotItineraryRoute();<br /> }</p> <p>
Let's step through this code briefly for an explaination:
Plotting Waypoints
After we have initialized the map we call plotItineraryRoute() to plot the points we want on the map. Let's look at a stripped down version to better understand things:
</p> <p>function plotItineraryRoute() {</p> <p> var start = "";<br /> var end = "";<br /> var waypts = [];</p> <p> var request = {<br /> origin: start,<br /> destination: end,<br /> waypoints: waypts,<br /> optimizeWaypoints: false,<br /> travelMode: google.maps.DirectionsTravelMode.DRIVING<br /> };</p> <p> directionsService.route(request, function(response, status) {<br /> if (status == google.maps.DirectionsStatus.OK) {<br /> directionsDisplay.setDirections(response);<br /> }else{// if (status == google.maps.DirectionsStatus.OK) {<br /> //error from google<br /> }<br /> });</p> <p>}</p> <p>
The free version of the API only allows 10 points to be plotted at once. You can see in the request object that there is an origin, destination, and waypoints property. The origin and destination count as 2 of the 10 points you can pass the API, so the waypoints array can only contain 8 elements. Google does have a paid premium service which allows for more points per call. For more information check Google's documentation and look for the status code "MAX_WAYPOINTS_EXCEEDED."
Waypoints Data
You can see that the origin point and destination point are empty. "waypoints" is also an empty array. So let's take a look at how to get data into these variables. For our solution we were storing point data in html elements, so we looped through those elements to assign values to these variables. How you get data into these variables is not important. How it's formatted is.
</p> <p> $('.map-element').each(function(index) {<br /> var points=$(this).attr('latitude')+","+$(this).attr('longitude');<br /> if(index==0){<br /> start=points;<br /> }else if(index==(len-1)){<br /> end=points;<br /> }else{<br /> if(wayptCounter<wayptLimit){<br /> waypts.push({<br /> location:points,<br /> stopover:true<br /> });<br /> wayptCounter++;<br /> }<br /> }<br /> });</p> <p>
There are two ways the API receives point data:
So either of these formats are acceptable for point data. You can see what's pushed into the waypt array is an object with two properties: location (a point formatted with one of the two options above) and stopover.
Customizing Rendering
We wanted to add a custom icon for each map point, so we hid the markers with following code:
</p> <p> var mapRendererOptions = {};</p> <p> var markerOptions ={};<br /> markerOptions.visible=false;</p> <p> var polylineOptions={};<br /> polylineOptions.strokeColor="#438391";<br /> polylineOptions.strokeOpacity=.6;<br /> polylineOptions.strokeWeight=4;</p> <p> //now assign to map render options<br /> mapRendererOptions.markerOptions=markerOptions;<br /> mapRendererOptions.polylineOptions=polylineOptions;</p> <p> //now set renderer<br /> directionsDisplay.setOptions(<br /> new google.maps.DirectionsRenderer(mapRendererOptions));</p> <p>
Now that we have marker options turned off, we need to go through each "leg" of the response object and place a custom marker on the map. We stored some additional metadata about each point in an array called "intineraryArray." We'll use this array as a holder of all point, but more important it contains the "user friendly" display name of the point. We don't want the user seeing "3499 Downing Rd, Denver, Colorado" - we want them to see "Aten's Office." Obviously the Google API doesn't have this display title.
</p> <p>var route = response.routes[0];<br /> var legs = response.routes[0].legs;</p> <p>for (i=0;i<legs.length;i++) {<br /> itineraryArray[i].address=legs[i].start_address;<br /> itineraryArray[i].duration=legs[i].duration.text;</p> <p> var altLineBreak="\n";</p> <p> if(i==(legs.length-1)){<br /> itineraryArray[i+1].address=legs[i].end_address;<br /> createMarker(legs[i].start_location,itineraryArray[i].label+<br /> altLineBreak+itineraryArray[i].address, i,<br /> "blue", markersItineraryArray);<br /> createMarker(legs[i].end_location,itineraryArray[i+1].label+<br /> altLineBreak+itineraryArray[i+1].address, i+1,<br /> "blue", markersItineraryArray);<br /> }else{<br /> createMarker(legs[i].start_location,itineraryArray[i].label+<br /> altLineBreak+itineraryArray[i].address, i,<br /> "blue", markersItineraryArray);<br /> }<br /> }</p> <p>
So for each leg it fires "createMarker." Here is the createMarker function:
</p> <p>function createMarker(latlng, label, index, color, markerArray) {<br /> var markerNumber="_"+(index+1);<br /> if(index==9){<br /> markerNumber="";<br /> }</p> <p> var marker = new google.maps.Marker({<br /> position: latlng,<br /> map: map,<br /> clickable: true,<br /> icon: new google.maps.MarkerImage(<br /> '/sites/all/modules/custom/trip_planner/img/'<br /> +color+'_marker'+markerNumber+'.png'),<br /> title: label<br /> });</p> <p> google.maps.event.addListener(marker, 'click', function() {<br /> //BLank click handler<br /> });<br /> }</p> <p>
Mapping that Makes Sense
The result is a powerful mapping tool that let's users plot destination points (points of interest) and their current itinerary, all on one map. The custom markers help distinguish which points belong to which lists.