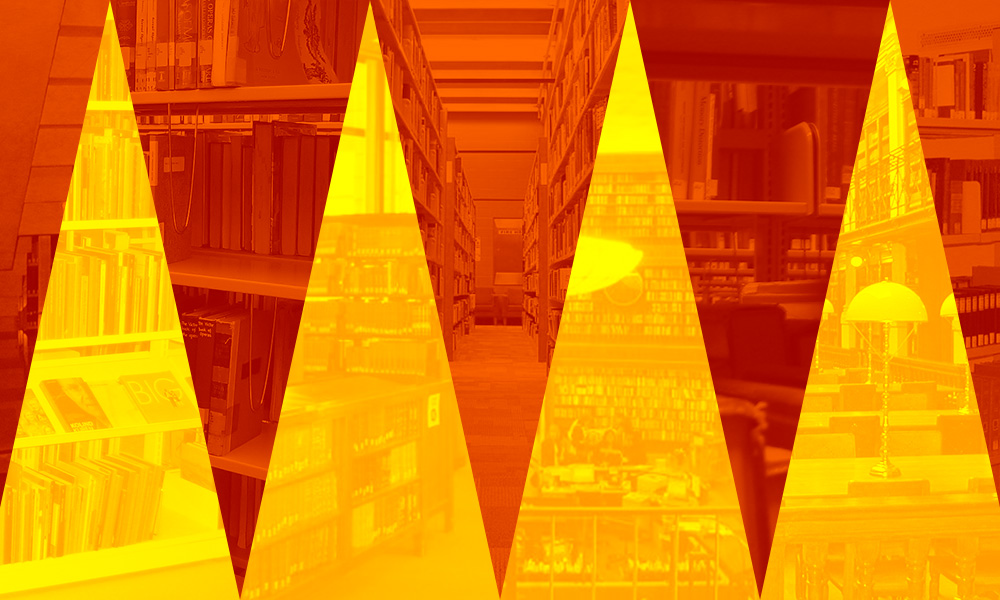
As a front-end developer, I often need to add JavaScript libraries to a project for front-end interaction. With most things Drupal, there are many different ways to go about adding these libraries, though some can be said to be more stylish than others. An approach that I’ve become particularly fond of utilizes a contributed module called Libraries API, which gives Drupal developers a consistent way to add libraries of all sorts.
To be entirely clear, a library is a bundle of code, often times CSS and JS, though it may be a bundle of PHP, or something else entirely. It's usually agnostic in the sense that the library isn’t targeted to Drupal applications.
In the past, I’ve added JS libraries directly to my theme, and then added the script to a page with drupal_add_js(). This means that the library is confined to the theme. If I want to use the library in a custom module as well, I have to reliably know the name of the theme, as that’s how drupal_get_path() works. That’s just straight-up bad practice, as it doesn't lend itself to modularity or reusable code.
Another method for adding JS libraries is the use of contributed modules named for the JS libraries they implement. The downside is that these JS libraries are often configured via the Drupal UI and frequently limit the configuration options of the library, or they are coupled to an outdated version of the library. In most cases, a requirement for these modules is that the library be added via Libraries API anyways.
By contrast, If I take the time to write a few extra lines of code and implement the library myself, I can ensure that it's available to other modules or themes in the project. This allows me to interact with the library the way the original author intended.
Working with Libraries API is fairly straightforward. As with all things, it’s a good idea to read the full documentation, which can be found at https://drupal.org/node/1342238, but you can get rolling with just a few lines of code.
$ drush dl libraries
Next, create a directory at sites/all/libraries. If you're not using Drush, download the module from drupal.org/project/libraries, and add it to the contrib modules directory.
<br /> /**<br /> * Implements hook_libraries_info().<br /> */<br /> function MYMODULE_libraries_info() {<br /> $libraries = array();<br /> $libraries['flexslider'] = array(<br /> 'name' => 'FlexSlider',<br /> 'vendor url' => 'http://flexslider.woothemes.com/',<br /> 'download url' => 'https://github.com/woothemes/FlexSlider/zipball/master',<br /> 'version arguments' => array(<br /> 'file' => 'jquery.flexslider-min.js',<br /> // jQuery FlexSlider v2.1<br /> 'pattern' => '/jQuery FlexSlider v(\d+.+\d+)/',<br /> 'lines' => 2,<br /> ),<br /> 'files' => array(<br /> 'js' => array(<br /> 'jquery.flexslider-min.js',<br /> ),<br /> ),<br /> );</p> <p> return $libraries;<br /> }<br />
One gotcha that seriously gotcha'd me is the necessity of providing a value for either version argument or version callback. The documentation for hook_libraries_info() says that both are optional, but if at least one isn't provided, the library isn't available to load. If you're not concerned about the version of the library, you can use a short-circuit function for the version callback:
<br /> /<strong><br /> * Implements hook_libraries_info().<br /> */<br /> function MYMODULE_libraries_info() {<br /> $libraries = array();<br /> $libraries['my_library'] = array(<br /> // Etc etc.<br /> 'version callback' => 'short_circuit_version',<br /> );</p> <p> return $libraries;<br /> }</p> <p>/</strong><br /> * Short-circuit the version argument.<br /> */<br /> function short_circuit_version() {<br /> return TRUE;<br /> }<br />
<br /> /<strong><br /> * Implements hook_preprocess_views_view().<br /> */<br /> function MYTHEME_preprocess_views_view(&$vars) {</p> <p> // Uncomment the lines below to see variables you can use to target a view.<br /> // This requires http://drupal.org/project/devel to be installed.<br /> // dpm($vars['view']->name, 'view name');</p> <p> // Hook view id specific functions.<br /> // This is a super neato trick.<br /> if (isset($vars['view']->name)) {<br /> $function = 'preprocess_views_view__' . $vars['view']->name;<br /> if (function_exists($function)) {<br /> $function($vars);<br /> }<br /> }<br /> }</p> <p>/</strong><br /> * Implements preprocess_views_view__VIEW().<br /> */<br /> function preprocess_views_view__YOURHOOK(&$vars) {<br /> $display_id = $vars['display_id'];<br /> $classes = &$vars['classes_array'];<br /> $title_classes = &$vars['title_attributes_array']['class'];<br /> $content_classes = &$vars['content_attributes_array']['class'];</p> <p> // Uncomment the lines below to see variables you can use to target a view.<br /> // This requires http://drupal.org/project/devel to be installed.<br /> // dpm($vars['view']->name, 'view name');</p> <p> switch ($display_id) {</p> <p> // Call flexslider scripts.<br /> case 'page':<br /> libraries_load('flexslider');<br /> break;<br /> }<br /> }<br />
$('.slideshow').flexslider({
animation: "slide",
controlNav: false,
namespace: 'slide-',
selector: '.slide-list > .slide-list-item'
});
}); // end window.load
})(jQuery);
As you can see, Libraries API allows for a much more elegant and scaleable way of managing JavaScripts.